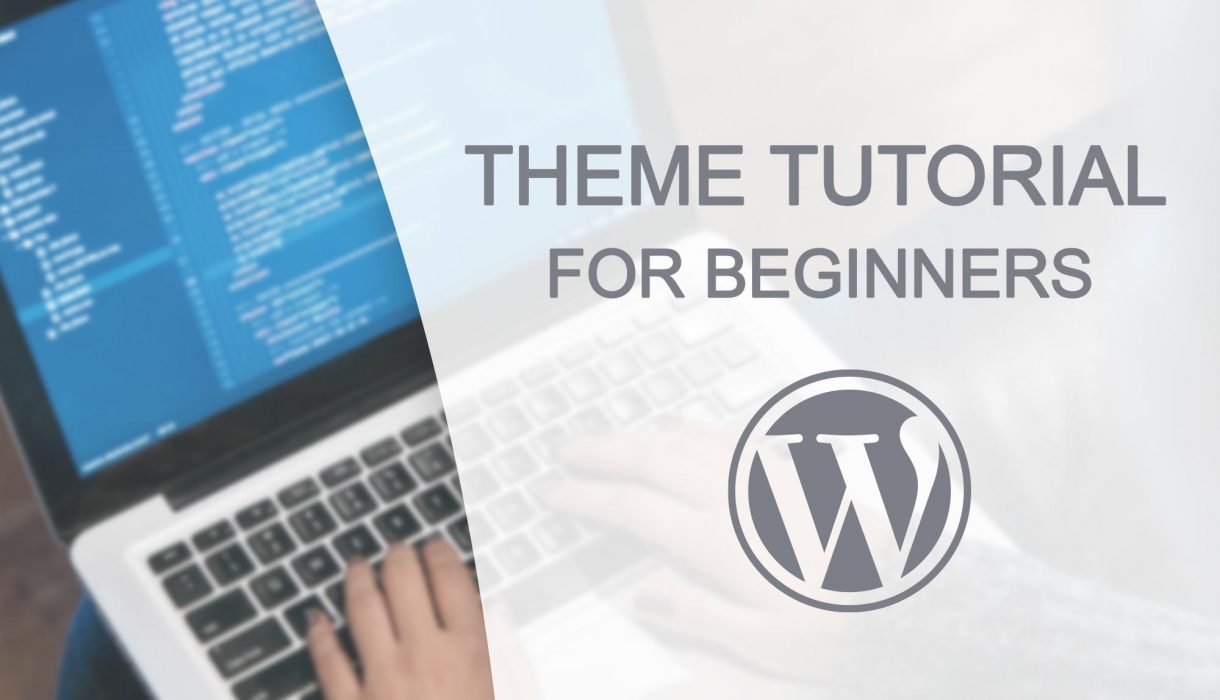
This lesson of the WordPress theme tutorial for beginners continues by adding the basic, required code for theme setup. We learn to add theme supports, and as a result of that WordPress’ featured image functionality gets activated. In this lesson we’ll also fix the frontpage title by applying our first filter.
We will work mostly in functions.php
file which we added in the previous lesson. At the end we will add code for outputting featured images inside our templates.
Theme Setup
All themes need some code for setting it up by telling WordPress to activate certain functionality. This includes activating menus, widgets, translation, and so forth. This is commonly done in a “setup theme function”, usually hooked onto a conveniently named hook after_setup_theme
. For some specific things we need to use the WordPress initializing hook called init
.
Writing necessary theme setup code for after_setup_theme
Let’s set up such a “setup theme function” in our functions.php
, hooked to after_setup_theme
:
functions.php
add_action('after_setup_theme', 'wptutorial_setup_theme'); function wptutorial_setup_theme() { }
Inside this function we will add a bunch of common and useful setup functions for WordPress themes. Please place all the following code pieces inside your setup function.
First we need to define a maximum-width of images and embeds (such as videos). This makes sure when authors insert large images in posts, the images keep within a certain width. I set it to 840 pixels (because I want room for sidebar), but you can adjust this to your liking.
$GLOBALS['content_width'] = 840;
We should also inform WordPress that the theme is translatable and where it can look for translation files. I will go into translation of WordPress theme in detail in next step in this theme tutorial series.
load_theme_textdomain('wptutorial', get_stylesheet_directory() . '/lang');
Note the use of a new function to get your theme directory, get_stylesheet_directory()
. This is very similar to get_stylesheet_directory_uri()
we encountered in the last step, but here we need the relative path, not the URL.
Then we need to add some “theme supports”, which is activating WordPress functionality which is not activated as default.
add_theme_support('automatic-feed-links'); add_theme_support('post-thumbnails'); add_theme_support('html5', [ 'search-form', 'comment-form', 'comment-list', 'gallery', 'caption', ]); add_theme_support('custom-logo', [ 'height' => 100, 'width' => 300, 'flex-width' => true, 'flex-height' => false, ]); add_theme_support('customize-selective-refresh-widgets');
That was a whole bunch of add_theme_support
calls! As the name implies, add_theme_support
tells WordPress to activate features that is not activated by default. For example featured images (‘post-thumbnails’), support for HTML5 tags, and WordPress’ custom logo feature in Customizer (adjust the desired logo image if you wish!).
There are many more cool features, so I encourage you to skim through the documentation for add_theme_support. For example there’s a fairly new one, title-tag
, which handles the title tag. I’ve left it out in this tutorial as we’ve already added the title tag in header.php
manually.
Finally we add a few add_theme_support
for the new Gutenberg in WordPress. We’ll add support for wide sections, activating block styles functionality and support for responsive embeds:
add_theme_support('wp-block-styles'); add_theme_support('align-wide'); add_theme_support('responsive-embeds');
The final result
Here is the final setup function in our functions.php
:
functions.php
add_action('after_setup_theme', 'wptutorial_setup_theme');
function wptutorial_setup_theme() {
$GLOBALS['content_width'] = 840;
// Make your theme ready for translation
load_theme_textdomain('wptutorial', get_stylesheet_directory() . '/lang');
// Add theme support for WordPress functionality
add_theme_support('automatic-feed-links');
add_theme_support('title-tag');
add_theme_support('post-thumbnails');
add_theme_support('html5', [
'search-form',
'comment-form',
'comment-list',
'gallery',
'caption',
]);
add_theme_support('custom-logo', [
'height' => 100,
'width' => 300,
'flex-width' => true,
'flex-height' => false,
]);
add_theme_support('customize-selective-refresh-widgets');
// Add theme support for Gutenberg specific functionality
add_theme_support('wp-block-styles');
add_theme_support('align-wide');
add_theme_support('responsive-embeds');
}
After saving you should now be able to add featured images to posts in admin! Let’s add one more theme fix in our functions.php
before we add featured images to our templates; remember that wp_title
in our header.php
by default can’t echo out anything on frontpage? Let’s fix that.
Adding a filter to wp_title
Let’s add our first proper filter modifying function, using add_filter()
. The filter we will hook onto has the same name as the function we used for WordPress to dynamically fetch the page title; wp_title
. What we will fix is making sure the title is not empty on the frontpage – which is default in WordPress. When on the frontpage we want it to be populated with the WordPress site’s name.
Knowing when to customize the title
We only want to customize the title variable if we are at the front page. For all other pages we want to leave it alone (just return it as it is). But how do we know when we are at the front page?
We can take advantage of WordPress’ conditional tags, which is a whole bunch of useful functions that returns true or false based on a condition. These are most commonly used to ask if we are at a certain page or template. We can simply ask “Are we on frontpage?”. If this method returns true, only then we will modify the title variable.
Getting the WordPress site’s name
Now we know what filter to use and how to make sure we only change the title if we are at the right place. But what should the title be? Usually the page title for the front page would be your WordPress site’s name. But how do we get that information?
For retrieving information about the current WordPress installation we use the bloginfo()
function. As parameter we ask to return the site name, which is done by setting the parameter to ‘name
‘. And because this is a filter and we need to return it, not echo it, we use its sister method get_bloginfo()
. Calling bloginfo()
will always echo output.
functions.php
add_filter('wp_title', 'wptutorial_title_filter'); function wptutorial_title_filter($title) { if (is_front_page()) { return get_bloginfo('name'); } return $title; }
If you refresh your frontpage, you should see that your site name appears in the <title>
tag (and in the browser tab)! Our filter affects only the frontpage, so any other pages such as single post, should still return the same as before; the post title.
Outputting featured images in template files
Earlier when we set up the theme supports we added support for featured images. With this activated we can now output these images in our templates. Let’s do that now!
Open up index.php
and find a place for them inside the loop. I chose to place it after the title, but it’s up to you where you want them. You use the function the_post_thumbnail()
to output the image. It’s recommended to first check if the post even has set a featured image, which we do by using has_post_thumbnail()
.
index.php
...
<h2><a href="<?php the_permalink(); ?>" title="<?php the_title(); ?>"><?php the_title(); ?></a></h2>
<?php if (has_post_thumbnail()) {
the_post_thumbnail();
} ?>
<?php the_excerpt(); ?>
...
If your post doesn’t have a featured image, you will see nothing being output after the title. However if you set a featured image on one of your posts, the_post_thumbnail()
would output an <img>
tag with the chosen image. This function accepts some additional parameters. For example you can define which image size you want to use, and control the attributes added onto the image. As usual I encourage you to take a quick look at the documentation and adjust to your liking.