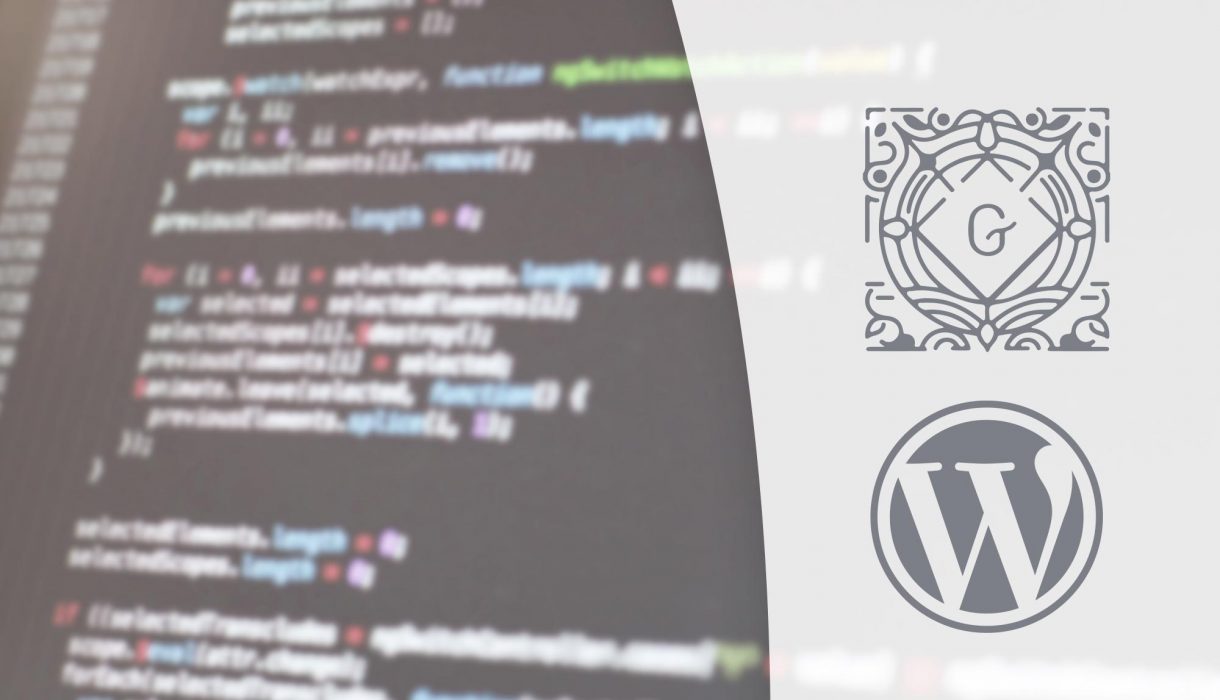
A look into WordPress Gutenberg’s new block patterns, in the eyes of a developer. We’ll look into what they are, what they can be used for, and a deeper look into how to write code for them.
Block patterns and their use
Block patterns were introduced in WordPress 5.5 (August 11th, 2020). They are a new feature in the block inserter to easier insert a predefined configuration of multiple blocks. Theme or plugin developers can define a group of blocks, how they are nested, their content and their attributes, and end-users can insert this with a simple one-click operation. The idea is that end-users no longer need to manually build complex layouts and all their custom settings, and nesting them in proper containers for blocks they use often.
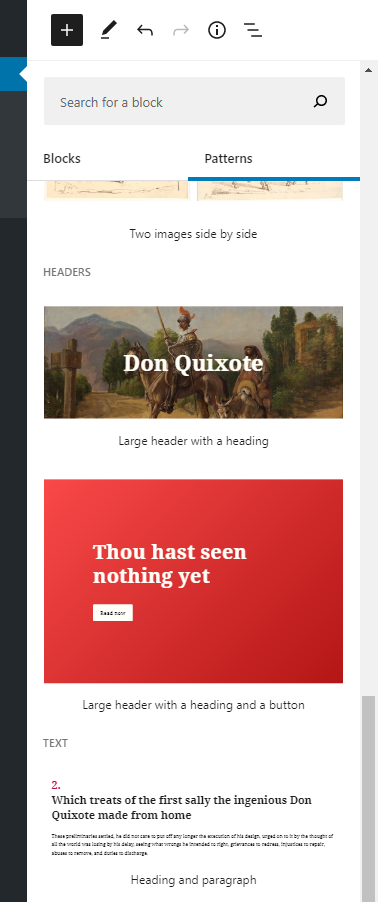
Once a block pattern is added to the editor, the blocks do not know that they were added through a block pattern. They are added as normal blocks allowing users to tweak their content and settings. It’s basically a shortcut of adding a configuration of multiple blocks.
The idea is a good one! Not all end-users are comfortable crafting a large structure of nested blocks in the editor in order to make it look perfect. However so far this feature is mostly a benefit for developers as end-users cannot create their own patterns. For users’ own customized blocks they need to stick with using reusable blocks. But especially for theme developers, block patterns let them truly show the end-users the best configuration of blocks that works well in the theme.
WordPress has added a new theme support for block patterns: 'core-block-patterns'
. Core will since 5.5.0 automatically run a add_theme_support('core-block-patterns')
so you don’t need to do this in your theme.
Since all the block pattern functions only exists in a very new WordPress version it’s a good idea to check for its existence first, so you don’t break sites with older WordPress versions. In all the code examples below I’ve done just that.
WordPress 5.5 ships with 9 predefined block patterns (see list below). Block patterns are displayed in pattern categories (core adds 5 categories). Block pattern categories work like post categories; you can put a block pattern in multiple categories. Theme and plugin developers can register their own block patterns and block pattern categories, as well as unregistering the ones in core. So let’s take a closer look at how!
Block patterns and block pattern categories included in WordPress 5.5
The block patterns shipped in WordPress 5.5 are as follows (the namespace and slug ID to each pattern are displayed in paranthesis):
- Two buttons (‘
core/two-buttons
‘) - Three buttons (‘
core/three-buttons
‘) - Two columns of text (‘
core/text-two-columns
‘) - Two columns of text with images (‘
core/text-two-columns-with-images
‘) - Three columns of text with buttons (‘
core/text-three-columns-buttons
‘) - Two images side by side (‘
core/two-images
‘) - Large header with a heading (‘
core/large-header
‘) - Large header with a heading and a button (‘
core/large-header-button
‘) - Quote (‘
core/quote
‘)
The categories added in WordPress 5.5 are (their slug IDs are displayed in paranthesis):
- Buttons (‘
buttons
‘) - Columns (‘
columns
‘) - Gallery (‘
gallery
‘) - Headers (‘
header
‘ – note no ‘s’ at the end) - Text (‘
text
‘)
Unregistering block patterns
You can unregister block patterns using the function unregister_block_pattern()
. Just provide a string with the block pattern to remove as parameter. Refer to the overview above for core block patterns. Run this function inside a function hooked to the init
action.
An example of unregistering the core block patterns “Two buttons” and “Three buttons”:
add_action('init', function() { if (!function_exists('unregister_block_pattern')) { return; } unregister_block_pattern('core/two-buttons'); unregister_block_pattern('core/three-buttons'); });
Registering block patterns
Registering a new block pattern is done by using the function register_block_pattern()
. It accepts two parameters; the first is a string of an unique name for your pattern including namespace. The second is an array of settings for your block pattern.
Tip: Defining a block pattern requires you to provide raw HTML content for your block configuration. I don’t recommend manually typing this out as this will easily cause invalid block conflicts. Instead go in the editor and set up the blocks as you wish to have them in your pattern. Then click the “dot menu” in the toolbar of the parenting block and click “Copy”. After this you can go back into your code editor and paste (Ctrl+V). This gives you the raw HTML to the configuration you copied. Use the code editor’s functionality to replace all newlines with \n
and make sure to escape quotes properly.
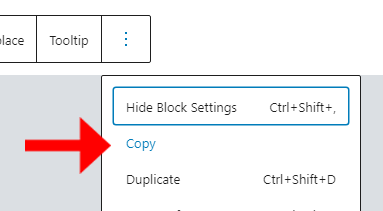
The properties for your block pattern are as follows (second argument array):
title
(requried): The displayable name of your block patterncontent
(required): RAW HTML of block configurationdescription
: Description for your block pattern. Is visually hiddencategories
: An array of categories to add this block pattern in. If you don’t provide this property the block will be placed in a “Uncategorized” block pattern category.keywords
: An array of keywords that can help users find your pattern while searchingviewportWidth
: Provide an integer of the block pattern width in the inserter. Affects only the preview in the inserter.
Here is an example of registering a block pattern that consists of a fullwidth Cover block that has a background color, that contains a center-aligned Heading block with a specific text color, and a center-aligned paragraph of a specific text color:
add_action('init', function() { if (!function_exists('register_block_pattern')) { return; } register_block_pattern('awp/standard-cta-section', [ 'title' => __('Standard CTA Section', 'awp'), 'keywords' => ['cta'], 'categories' => ['header'], 'viewportWidth' => 1000, 'content' => "<!-- wp:cover {\"customOverlayColor\":\"#dadee2\",\"align\":\"full\"} -->\n<div class=\"wp-block-cover alignfull has-background-dim\" style=\"background-color:#dadee2\"><div class=\"wp-block-cover__inner-container\"><!-- wp:heading {\"align\":\"center\",\"level\":1,\"style\":{\"color\":{\"text\":\"#414446\"}}} -->\n<h1 class=\"has-text-align-center has-text-color\" style=\"color:#414446\">Section title</h1>\n<!-- /wp:heading -->\n\n<!-- wp:paragraph {\"align\":\"center\",\"style\":{\"color\":{\"text\":\"#414446\"}}} -->\n<p class=\"has-text-align-center has-text-color\" style=\"color:#414446\">Write your text here.</p>\n<!-- /wp:paragraph --></div></div>\n<!-- /wp:cover -->", ]); });
Registering block pattern categories
Developers can also register custom block pattern categories. This is done with the function register_block_pattern_category()
. It accepts two parameters; first a string of the category slug, and second an array of properties. As of right now only one property is supported in the second argument; label
for the readable name of the category.
Below is an example of registering a custom block pattern category:
add_action('init', function() { if (!function_exists('register_block_pattern_category')) { return; } register_block_pattern_category('awp-patterns', ['label' => __('AWhitePixel Block Patterns', 'awp')]); });
With this you can add ‘awp-patterns’ to the ‘categories
‘ argument to register_block_pattern()
. Keep in mind that if a category has no block patterns registered to it, the category will not be displayed in the block inserter. You will need to add at least one block pattern in this category to make it appear.
Unregistering block pattern categories
Finally there is a function unregister_block_pattern_category()
to unregister a block pattern category. Provide the category slug as parameter. Refer to the overview of block pattern categories above for core categories and their slugs.
Don’t forget that block pattern categories without any block patterns assigned to it will not be visible in the block inserter. So if you unregister all block patterns assigned to a category the category itself will no longer be visible and you don’t necessarily need to unregister the category. Any block patterns that are only assigned to the category you are removing, will be moved into a “Uncategorized” category.
Here is an example of unregistering the core block pattern category ‘buttons’:
add_action('init', function() { if (!function_exists('unregister_block_pattern_category')) { return; } unregister_block_pattern_category('buttons'); });
Conclusion
The new block pattern feature in WordPress Gutenberg is definitely a step closer to making the block editor work more as a page builder. In my opinion the downside is that end-users cannot create their own patterns. But it’s a good function for theme developers as to allow end-users to easily set up block configurations and layouts that work well in the theme. This is a brand new feature and it’ll probably evolve and be improved in the near future! I for one, cannot wait!